Your REST API should have been HATEOAS, but it isn't
It's not hard to see why adding HAL reduces the need to overly document your API. HAL solution is not just more elegant, but it's also something that induces less frontend code, more flexibility, less stuff braking when you decide to change something
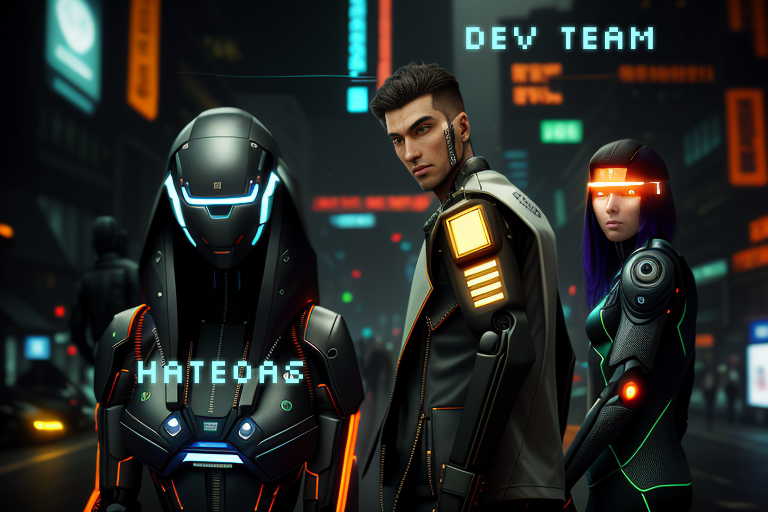
Okay, so this a weird sentence right at the start, let's take a step back and summarize what on earth I'm talking about.
HATEOAS+HAL
A.k.a. Hypermedia as the engine of application state. This un-decodable, unpronounceable shorthand is a REST application architecture (or more accurately, a set of constraints), that I could best describe as MSG, just for your APIs. It sprinkles on a lot of additional information that in the end makes navigating the API much easier (even for other programs). But it's only a concept on its own. Hypertext Application Language or HAL is one manifestation of the HATEOAS concept.
Links and discovery
Let's look at an example on a HAL response:
{
"_links": {
"self": { "href": "/orders" },
"next": { "href": "/orders?page=2" },
"find": { "href": "/orders{?id}", "templated": true }
},
"_embedded": {
"orders": [{
"_links": {
"self": { "href": "/orders/123" },
"basket": { "href": "/baskets/98712" },
"customer": { "href": "/customers/7809" }
},
"total": 30.00,
"currency": "USD",
"status": "shipped"
},{
"_links": {
"self": { "href": "/orders/124" },
"basket": { "href": "/baskets/97213" },
"customer": { "href": "/customers/12369" }
},
"total": 20.00,
"currency": "USD",
"status": "processing"
}]
},
"currentlyProcessing": 14,
"shippedToday": 20
}
Okay, so there's a lot to untangle here. First of all, we have 2 main objects:
We have _links
on the top level, which is a collection that can contain the main places that can be navigated from here. You can already see that you don't really need to worry about the pagination logic on your frontend, because as long as you see the next
link you can be sure that there is a next page which you can immediately query. There is a reference to find a specific order as well.
The other bit is the _embedded
section which is your data returned, just like your normal REST endpoint would, except we also have links for each related object. You can use the self
links to go to that order straight or here the endpoint also returns the links to the related objects that you might want to go to instead, like the customer
or the basket
of that order.
And this is only the tip of the iceberg.
There's so much stuff you can augment your endpoint with, e.g. links can contain title
, name
, even information about deprecation
of that specific endpoint, and so much more. I highly recommend just glancing over the IETF draft document which I linked under references.
Your API is self-documenting now.
It's not hard to see why adding HAL reduces the need to overly document your API. Even if you have a fancy metaprogramming swagger/OpenAPI setup that uses your JSDoc/JavaDoc/Whatever to generate the API documentation you can't deny that this HAL solution is not just more elegant, but it's also something that induces less frontend code, more flexibility, less stuff braking when you decide to change something (because you can just change the _link
) and most importantly, much more dynamic than your hardcoded endpoints in the consumer side.
TLDR and conclusion:
You should build your REST APIs with HATEOAS+HAL architecture as there are just too many advantages and it's not really much effort to implement (Especially for you, Java/Spring guys!). Spread the word, there's no excuse and you should start shaming other developers for their plain boring REST APIs. This thing has been here with us for more than ten years and we should all do our APIs this way.
References:
